Lists are one of the fundamental data structures in Python, offering a versatile and powerful way to store and organize multiple elements. In Python, lists are mutable, ordered, and can contain elements of different data types. They provide flexibility for storing and manipulating data, making them indispensable for various programming tasks. This article will explore the concept of lists in Python, covering everything from their creation and initialization to accessing and manipulating list elements. We will also delve into common list operations, working with nested lists, list comprehension, and share some useful tips and best practices. Whether you are a beginner or an experienced Python developer, this article will serve as a comprehensive guide to mastering lists in Python.
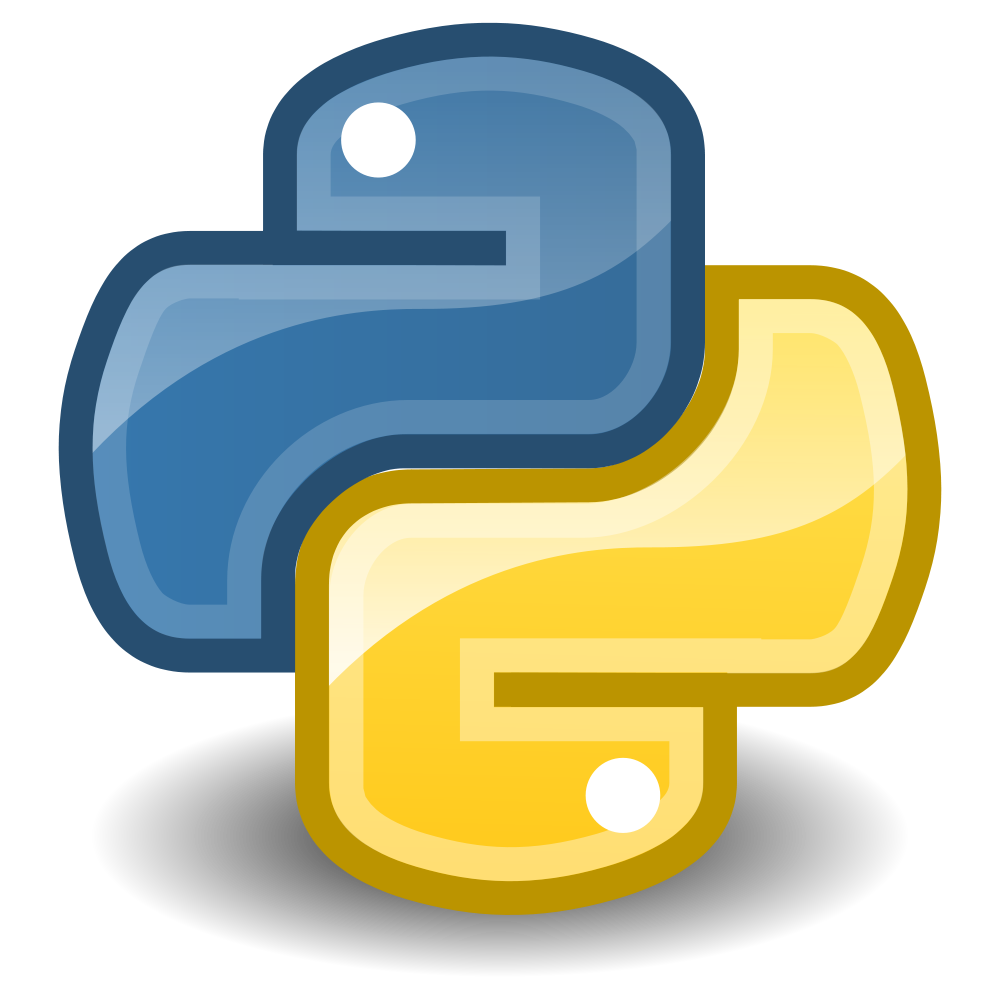
1. Introduction to lists in Python
1.1 What are lists?
Lists are one of the most versatile and commonly used data structures in Python. Simply put, a list is an ordered collection of items, where each item can be of any data type. Lists are enclosed within square brackets [] and each item is separated by a comma.
1.2 Why are lists important in Python?
Lists play a crucial role in Python programming because they allow us to store and manipulate multiple values in a single variable. Unlike other data types, lists are mutable, which means we can change, add, or remove elements from the list. This flexibility makes lists extremely useful for tasks like storing data, implementing algorithms, and organizing information.
2. Creating and initializing lists
2.1 Declaring a list
To create a list, we simply assign a set of values to a variable using the list notation. For example:
“`
fruits = [‘apple’, ‘banana’, ‘orange’]
“`
Here, we have created a list called “fruits” containing three strings.
2.2 Initializing an empty list
Sometimes we might want to create an empty list and fill it later. To initialize an empty list, we can use empty square brackets. For example:
“`
numbers = []
“`
Now we have an empty list called “numbers” ready to store numeric values.
2.3 Initializing a list with values
We can also initialize a list with specific values right from the start. To do this, we enclose the values within square brackets, separating each value with a comma. For example:
“`
scores = [90, 85, 95, 80]
“`
In this case, we have initialized a list called “scores” with four integers representing test scores.
3. Accessing and manipulating list elements
3.1 Indexing and slicing lists
To access individual elements of a list, we use indexing. The first element of a list has an index of 0, the second element has an index of 1, and so on. We can also use negative indexing to access elements from the end of the list. For example:
“`
fruits = [‘apple’, ‘banana’, ‘orange’]
print(fruits[0]) # Output: apple
print(fruits[-1]) # Output: orange
“`
Additionally, we can use slicing to access a specific range of elements in a list. Slicing is done using the colon (:) symbol. For example:
“`
numbers = [1, 2, 3, 4, 5]
print(numbers[1:4]) # Output: [2, 3, 4]
“`
3.2 Modifying list elements
Since lists are mutable, we can modify their elements by assigning new values to specific indices. For example:
“`
fruits = [‘apple’, ‘banana’, ‘orange’]
fruits[1] = ‘grape’
print(fruits) # Output: [‘apple’, ‘grape’, ‘orange’]
“`
Here, we have changed the second element of the list from “banana” to “grape”.
3.3 Adding and removing elements
To add elements to a list, we can use the append() method, which adds an item to the end of the list. For example:
“`
numbers = [1, 2, 3]
numbers.append(4)
print(numbers) # Output: [1, 2, 3, 4]
“`
To remove elements from a list, we can use methods like remove() and pop(). The remove() method removes the first occurrence of a specific value, while the pop() method removes an element at a specified index. For example:
“`
fruits = [‘apple’, ‘banana’, ‘orange’]
fruits.remove(‘banana’)
print(fruits) # Output: [‘apple’, ‘orange’]
numbers = [1, 2, 3, 4]
numbers.pop(2)
print(numbers) # Output: [1, 2, 4]
“`
4. Common list operations and methods
4.1 Finding the length of a list
To determine the number of elements in a list, we can use the len() function. For example:
“`
fruits = [‘apple’, ‘banana’, ‘orange’]
print(len(fruits)) # Output: 3
“`
4.2 Concatenating lists
We can combine two or more lists together using the concatenation operator (+). This creates a new list containing all the elements of the original lists. For example:
“`
list1 = [1, 2, 3]
list2 = [4, 5, 6]
combined_list = list1 + list2
print(combined_list) # Output: [1, 2, 3, 4, 5, 6]
“`
4.3 Sorting and reversing lists
Python provides methods like sort() and reverse() to sort and reverse the order of elements in a list, respectively. For example:
“`
numbers = [4, 2, 1, 3]
numbers.sort()
print(numbers) # Output: [1, 2, 3, 4]
fruits = [‘apple’, ‘banana’, ‘orange’]
fruits.reverse()
print(fruits) # Output: [‘orange’, ‘banana’, ‘apple’]
“`
So there you have it, a comprehensive guide to working with lists in Python. Lists are a powerful tool in your programming arsenal, allowing you to efficiently store, access, and manipulate collections of data. With this understanding, you’ll be well-equipped to tackle a wide range of programming tasks using Python’s versatile lists. Happy coding!
5. Working with nested lists
Nested lists can be a powerful tool when it comes to organizing and manipulating data in Python. Essentially, a nested list is a list that contains other lists as its elements. In this section, we’ll explore how to create, access, modify, and iterate through nested lists.
5.1 Creating and accessing elements in nested lists
Creating a nested list is as simple as assigning a list to another list. For example:
“`
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
“`
To access elements within a nested list, we use a combination of square brackets to indicate the level of nesting. For example:
“`
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
print(nested_list[1]) # [4, 5, 6]
print(nested_list[0][2]) # 3
“`
5.2 Modifying and iterating through nested lists
Modifying elements in a nested list follows the same principles as modifying elements in a regular list. We can use square brackets to access specific elements and assign new values to them. For example:
“`
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
nested_list[0][1] = 10
print(nested_list) # [[1, 10, 3], [4, 5, 6], [7, 8, 9]]
“`
When it comes to iterating through a nested list, we can use nested loops. The outer loop iterates over the main list, while the inner loop iterates over the sub-lists. For example:
“`
nested_list = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
for sublist in nested_list:
for element in sublist:
print(element)
“`
This will print each element in the nested list on a separate line.
6. List comprehension in Python
List comprehension is a concise and elegant way to create lists in Python. It allows us to generate a new list by applying an expression to each element of an existing list (or other iterable). In this section, we’ll dive into the what, how, and why of list comprehension.
6.1 What is list comprehension?
In simple terms, list comprehension is a compact way to create a new list based on an existing list (or other iterable) while applying some modification or filtering. It combines the creation and transformation steps into a single line of code, making it both powerful and efficient.
6.2 Syntax and examples
The basic syntax for list comprehension is as follows:
“`
new_list = [expression for item in iterable if condition]
“`
Here’s an example that demonstrates how to use list comprehension to create a new list of squared numbers:
“`
numbers = [1, 2, 3, 4, 5]
squared_numbers = [x**2 for x in numbers]
print(squared_numbers) # [1, 4, 9, 16, 25]
“`
6.3 Benefits and use cases
List comprehension offers several benefits, including improved readability, reduced code length, and increased efficiency. It’s particularly useful when you need to perform a simple transformation or filtering operation on an existing list.
Some common use cases for list comprehension include generating new lists based on existing ones, filtering out specific elements, converting between data types, and performing mathematical calculations.
7. Useful tips and best practices for working with lists
Working with lists in Python is a fundamental skill that every programmer should master. In this section, we’ll cover some useful tips and best practices to help you make the most out of your list-related endeavors.
7.1 Naming conventions for lists
When naming lists, it’s important to choose descriptive names that convey their purpose or the type of data they contain. This makes your code more readable and helps future developers (including yourself) understand the intention behind the list.
For example, instead of naming a list “l”, consider using a more meaningful name like “student_names”.
7.2 Avoiding common pitfalls
One common mistake when working with lists is modifying a list while iterating over it. This can lead to unexpected behavior or even runtime errors. To avoid this, it’s recommended to create a copy of the list before iterating over it if you plan to modify its elements.
Another common pitfall is forgetting to use the `range()` function correctly when iterating over a list. Remember that the indices of a list start at 0, so you’ll need to adjust the range accordingly.
7.3 Optimizing list performance
If you’re dealing with large lists or performance-critical operations, there are a few techniques you can employ to optimize your code. These include using list comprehension instead of traditional loops, leveraging built-in functions like `map()` and `filter()`, and using generator expressions instead of creating unnecessary lists.
Keep in mind that premature optimization is generally discouraged, as it can complicate your code and make it harder to maintain. Only optimize when necessary and after profiling your code to identify the bottlenecks.
8. Conclusion and further resources
In this article, we’ve covered the ins and outs of working with lists in Python. From nested lists to list comprehension, we’ve explored various techniques to make your list-related operations more efficient and enjoyable.
If you want to dive deeper into Python lists, there are plenty of resources available. Check out the official Python documentation, online tutorials and guides, or join Python communities and forums to engage with fellow developers. Happy coding!
FAQ
1. Can lists in Python contain elements of different data types?
Yes, lists in Python can contain elements of different data types. Unlike arrays in some other programming languages, Python lists do not have a fixed element type. This flexibility allows you to store integers, floats, strings, booleans, or even other lists within a single list.
2. What is the difference between indexing and slicing a list?
Indexing a list refers to accessing a specific element in the list by its position, starting from 0 for the first element. Slicing, on the other hand, allows you to extract a portion of a list by specifying a range of indices. For example, `my_list[2]` will retrieve the third element, while `my_list[1:4]` will extract elements from the second to the fourth element (excluding the fourth).
3. What are some common list operations and methods in Python?
Python provides a variety of operations and methods to work with lists. Some common operations include finding the length of a list with the `len()` function, concatenating lists with the `+` operator, and sorting lists using the `sort()` method. Additionally, there are methods such as `append()`, `remove()`, and `insert()` for adding, removing, and inserting elements into a list, respectively.
4. How can I optimize the performance of list operations?
To optimize the performance of list operations, it is recommended to use list comprehension instead of traditional loops whenever possible. List comprehension allows you to perform operations on lists in a more concise and efficient manner. Additionally, if you need to perform extensive data manipulations, consider using other data structures like arrays or pandas dataframes that are designed for faster computations and data processing.
Choose the right career path by identifying your skills & interests. Get online career counselling and career guidance
Important Links
- Python Course
- Machine Learning Course
- Data Science Course
- Digital Marketing Course
- Python Training in Noida
- ML Training in Noida
- DS Training in Noida
- Digital Marketing Training in Noida
- Winter Training
- DS Training in Bangalore
- DS Training in Hyderabad
- DS Training in Pune
- DS Training in Chandigarh/Mohali
- Python Training in Chandigarh/Mohali
- DS Certification Course
- DS Training in Lucknow
- Machine Learning Certification Course
- Data Science Training Institute in Noida
- Business Analyst Certification Course
- DS Training in USA
- Python Certification Course
- Digital Marketing Training in Bangalore
- Internship Training in Noida
- ONLEI Technologies India
- Python Certification
- Best Data Science Course Training in Indore
- Best Data Science Course Training in Vijayawada
- Best Data Science Course Training in Chennai
- ONLEI Group
- Data Science Certification Course Training in Dubai , UAE
- Data Science Course Training in Mumbai Maharashtra
- Data Science Training in Mathura Vrindavan Barsana
- Data Science Certification Course Training in Hathras
- Best Data Science Training in Coimbatore
- Best Data Science Course Training in Jaipur
- Best Data Science Course Training in Raipur Chhattisgarh
- Best Data Science Course Training in Patna
- Best Data Science Course Training in Kolkata
- Best Data Science Course Training in Delhi NCR
- Best Data Science Course Training in Prayagraj Allahabad
- Best Data Science Course Training in Dehradun
- Best Data Science Course Training in Ranchi